Best coding practices in Javascript
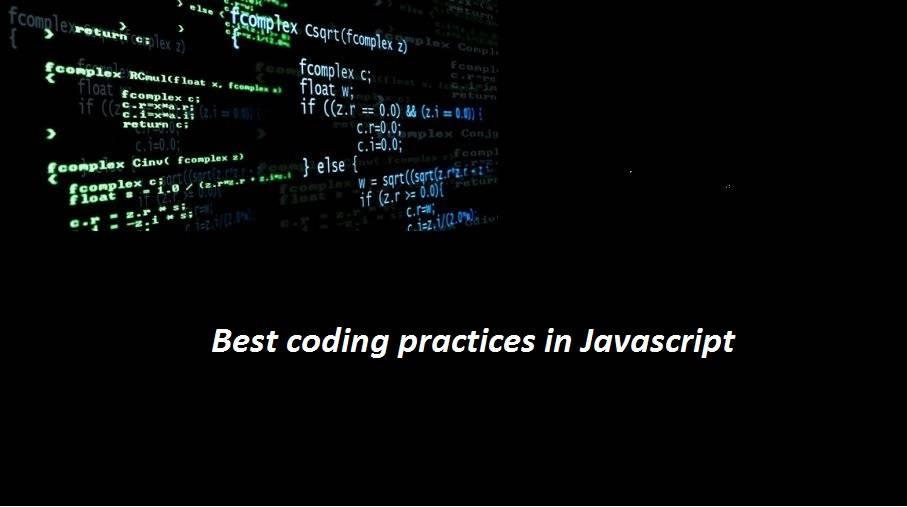
JavaScript is a powerful and most popular language for programming on Web and it is the most preferred one for real-time application development these days. There are many JavaScript frameworks available fashioned for specific type of application development and each has its own unique features. While developing an application using any of the programming languages we should always strive for standard coding practices which makes the code readable and standardized.
In JavaScript, we have some set of standards and best coding practices to follow, which will help your code keep clean and very proficient.
Here are some basic coding practices to follow while development.
Use === Instead of ==
JavaScript allows us to use two type of comparison operators `===` and `==`,
`===` This comparison operator will check for the type of operands and the value, if these two rules are valid then the comparison will pass else it will consider as failed.
`==` This comparison operator will just check for the value same, but not the type of the operands. So the case of values is same it will consider as passed. The cause of this comparison is for the long run of the code if the operand passes the value with a different type of variable type further arithmetic operations will be end-up with failed.
Eg:-
const a = 5; const b = ‘5’; if(a == b) { const c = a + b; console.log(‘c’, c); } Result :- c, 55
Early calling method
Javascript allows conditional based calling based on needs, if code supposes to run 1 line after the conditional statement it is good to use early calling method.
if (!someCondition) return ;
Else if we have more than one line code to run after the conditional statement.
if (!someCondition) { const x = ‘xyz’; callAnotherFunction(); }
Utilize JS Lint
JS Lint is a debugger written by Douglas Crockford, it allows to validate our code written. JS Lint is available as a plugin for different IDE.
“JSLint takes a JavaScript source and scans it. If it finds a problem, it returns a message describing the problem and an approximate location within the source. The problem is not necessarily a syntax error, although it often is. JSLint looks at some style conventions as well as structural problems. It does not prove that your program is correct. It just provides another set of eyes to help spot problems.”
– JSLint Documentation
Declare Variables Outside the Loop Statement
Use variables declaration outside the loop statement, when we use declaring variable in the loop statement it allocates memory for each time variable declare and which cause high usage of memory usage and app execution will be slower.
Must follow:
const x = 0; const element = document.getElementById(‘someId’); [‘a’,b’,’c’].forEach(function(item) { x = x + 1; element.innerHTML = item + ‘: ’ + x; });
Reduce Globals
By reducing the global variables using in the application will reduce the interactions with other widgets, plugins and working will be stable.
Usage:
const calc = { x: 1, y: 1, calculation: function() { return x * y; } }]; console.log(calc.x,calc.y,calc.calculation());
Comment Your Code
Usage of commenting the logics that we implemented in the application will be helpful for other developers can take look on it and grab the idea easily in the future run of the application.
// Looping the array and console the array key and value [1, 2, 3, 4].forEach(function(item, key) { console.log(‘item’, item, ‘key’, key); });
Use {} Instead of New Object()
There is a lot of ways to create an object in javascript application development.
Traditional:
var a = new Object(); a.firstname = “John”; a.lastname = “Snow”; function displayName() { return a.firstname + ‘ ’ + a.lastname; }
Better:
const a = { firstname: ‘John’, lastname: ‘Snow’, displayName: function() { return this.firstname + ‘ ’ + this.lastname; } };
Note the better way to create an empty object is:
const a = {};
Use [] Instead of New Array()
Same as Object defining, there is a lot of ways to define Array in javascript development.
Traditional:
var a = new Array(‘a’, ‘b’); a.push(‘c’);
Better:
const a = [‘a’,’b’]; a.push(‘c’);
Long list of variables to define
If there is a long list of variables to define in javascript development. Like all programming languages does javascript allow us to use define variables by separating variables by comma and omit variable type for each variable.
The way we use before:
var a = 1; var b = 2; var c = 3;
Better:
const a = 1, b = 2, c = 3;
Always, Always Use Semicolons
It’s a bad practice that we would use the semicolon while developing the application. Technically, all browsers will allow omitting semicolon while running the code, but when there is issue come up in the running application. It is hard to find out the problem for the bigger functions.
Bad practice:
var firstname = “John”; var lastname = “Snow”; function displayName() { return firstname + ‘ ’ + lastname }
Best practice:
const a = { firstname: “John”, lastname: “Snow”, displayName: function() { return this.firstname + “ ” + this.lastname; } }; console.log(“displayName”, a.displayName());
Immediate Invokable Function Expersion (IIFE) or Simply call it Self-Executable Functions
In javascript, we can create self-executable functions and write the code inside the self-executable function rather than calling each oftheme separately.
(function() { console.log(“Initiating the function call”); })();
Use ES6 coding standard
As we all know the current Node.JS versions is improved and added lot of modern language syntax supports in the latest stable version. In the latest stable version of Node.JS support, ES6 coding standard and ES6 coding standard reduce the line of codes to compile and easy to understand the code.
Traditional:
var a = new Array(); a[0] = new Object({ x: 1, y: 2 }); A[1] = new Object({ x: 2, y: 3 }); a.forEach(function(item){ console.log(“SUM”, item.x * item.y); });
Using ES6:
const a = [{ x: 1, y: 2 }, { x: 2, y: 3 }]; const sum =a.forEach((item) => console.log(“SUM”, item.x * item.y));
Use Loadash or UnderscoreJS for the development
Javascript libraries provide various features apart from the raw functions, which will reduce the complexity of the development and less execution time for the logic we implemented in the application.
Traditional:
var a = new Array(); a[0] = new Object({ x: 1, y: 2 }); A[1] = new Object({ x: 2, y: 3 }); var sum = new Array(); a.forEach(function(item){ sum.push(item.x * item.y); });
Better using UnderscoreJS:
const a = [{ x: 1, y: 2 }, { x: 2, y: 3 }]; const sum = _.map(a, (item) => item.x * item.y);
Know More About This Topic from our Techies
Other Blogs: