Getting Started with Gulp.js
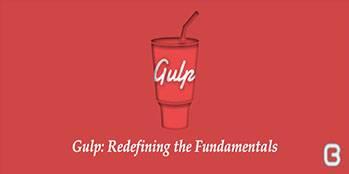
Gulp is a javascript task runner, a tool that helps with several tasks, when it comes to web development. Gulp is also a streaming build system. By using streams of nodejs, file manipulation is all done in memory, and a file isn’t written until it is said to do so. Gulp’s main focus is on speed, efficiency and simplicity. You code your own build process and use JavaScript as the language. There are nearly 800 plugins ready for Gulp.js, but even more Node.js modules can also be used to build the perfect build and development process for your needs.
Gulp is basically a tool for automating tasks like minification, compilation, unit testing, etc, which we do on a daily basis.
Installing Gulp
You need to have Node.js (Node) installed onto your computer before you can install Gulp.
When you’re done with installing Node, you can install Gulp by using the following command in the command line:
$ sudo npm install gulp -g
The npm install command used here uses Node Package Manager (npm) to install Gulp onto the computer.
The -g flag in this command tells npm to install Gulp globally onto the computer, which allows you to use the gulp command anywhere on your system.
You may need the extra sudo keyword in the command if administrator rights are needed to install Gulp globally.
Now that you have Gulp installed, let’s make a project that uses Gulp.
Creating a Gulp Project
From now on all the commands you’ll be running will be from the project’s root folder. The project needs a file called package.json. So create one in the project’s folder, the contents of the file should be something like:-
{ "name": "gulp", "version": "1.0.0", "devDependencies": { } }
you could generate the file by running npm init command from inside our project folder
This will prompt you to give values for certain parameters. You may add the values you want or just keep on pressing Enter key to complete the process with default values. This will create the package.json file.
Once the package.json file is created, we can install Gulp into the project by using the following command:
$ npm install gulp –save-dev
You’ll see that the sudo keyword isn’t required because we’re not installing Gulp globally, so -g is also not necessary. We’ve added –save-dev, which tells to add gulp as a dev dependency in package.json.
{ "name": "gulp", "version": "1.0.0", "devDependencies": { “gulp” : “^3.9.0” } }
And finally we’ll need a gulpfile.js in your project root that contains your tasks.
Writing Your First Gulp Task
The first step to using Gulp is to require it in the gulpfile.
var gulp = require('gulp');
The basic syntax of a gulp task is:
gulp.task('task-name', [dependent-tasks] ,function() { // Stuff here }); gulp.task('hello', function() { console.log('Hello Gulp'); });
We can run this task with gulp hello in the command line. You can run gulp command without the name of the task. In this case, gulp will check for the ‘default’ task.
gulp.task('default', [‘hello’]);
Now running gulp in command line will run the hello task.
Let’s start building a real task where we compile Sass files into CSS files.
Preprocessing with Gulp
We can compile Sass to CSS in Gulp with the help of a plugin called gulp-sass. Gulp-sass can be easily installed to the your project via the same npm command that we used for installing the gulp.
We’d also want to use the –save-dev flag to ensure that gulp-sass gets added to devDependencies in package.json.
$ npm install gulp-sass --save-dev
Add the following to our gulpfile.js
var gulp = require('gulp'); var sass = require('gulp-sass'); gulp.task('sass', function(){ gulp.src(['public/sass/*.scss']) .pipe(sass({outputStyle: 'compressed'})) .pipe(gulp.dest('public/css')); });
gulp.src tells the Gulp task what files to use for the task, while gulp.dest tells Gulp where to output the files once the task is completed.
Here we define a task to take all files with extension .scss from the sass folder, convert it to css, compress and write it to css folder.
Similarly, we can use the gulp-uglify plugin to minify the JavaScript files.
$ npm install gulp-uglify --save-dev
Modify the gulpfile.js to create a minjs task, which will take files from js folder, minfies and saves it.
var minify = require('gulp-uglify'); // THIS WILL TAKE ALL JS FILES INSIDE JS FOLDER AND ITS SUB FOLDERS, EXCEPT THE .MIN.JS FILES. MINIFY IT AND SAVE IT gulp.task('minjs', function(){ gulp.src(['public/js/**/*.js','!public/js/**/*.min.js']) .pipe(minify()) .pipe(gulp.dest('dist/js')); });
Watching a Task
Gulp provides us with a watch method that checks to see if a file was saved. This time there’s no need to install a plugin. The syntax for the watch method is:
// Gulp watch syntax gulp.watch('files-to-watch', ['tasks', 'to', 'run']);
If we want to watch all Sass files and run the sass task whenever a Sass file is saved, we just have to write:
gulp.watch([‘public/sass/*.scss’], [‘sass’]);
Modify the gulpfile.js to create a watch task
gulp.task('default', ['watch']); gulp.task('watch', function() { gulp.watch('public/sass/*.scss' , ['sass']); gulp.watch('public/js/**/*.js' , ['minjs']); });
If you ran the gulp command, you’ll see that Gulp starts watching immediately.
And that it automatically runs the sass task whenever you save a .scss file and minjs task whenever a js file is modified in the js folder.
Wrapping it up
I have walked you through the basics of Gulp and also highlighted the process of compiling Sass into CSS, minifying JS while watching files for changes at the same time. We can run this task with the gulp command in the command line.
As of now, the Gulp comes with hundreds of plugins and each of these plugins have a unique role to play in automating the different tasks that can be done via Gulp and eventually speed up the web development process.