Introduction to ReactJs with ES6
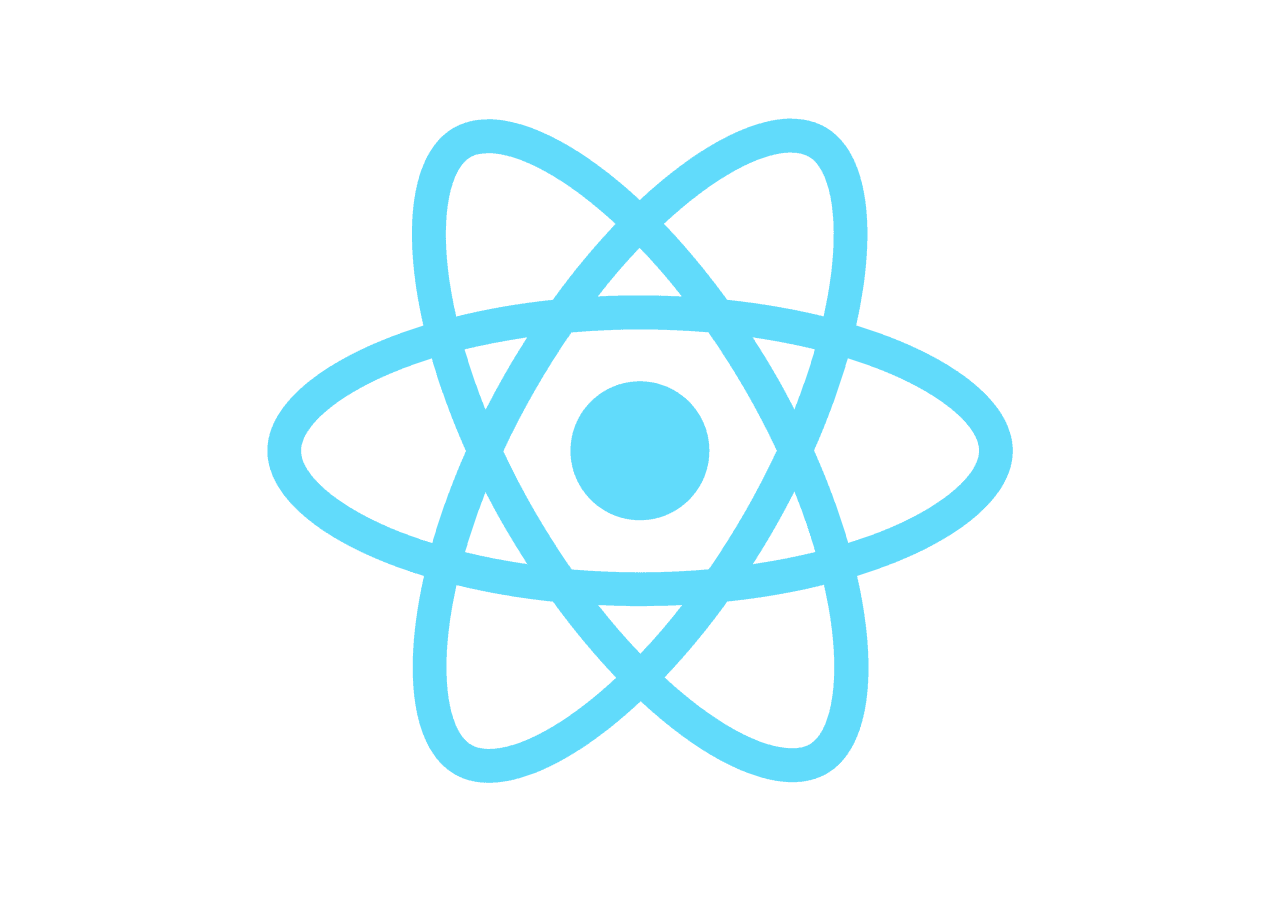
Web development is going like a supersonic these days, by the entries of various JavaScript technologies like ReactJs, AngularJs, NodeJs etc. Most of the area is covered by JavaScript, by their unique features and their performance, as well as JavaScript is very easy to use and manage. Introducing some features, related to the top-rated JavaScript framework ReactJs, including a very useful feature introduced by JavaScript called ES6(ECMAScript 2015).
Setting up React application
We can simply set up the react application in a few steps. Before that make sure you have the nodejs installed in your system. You can find the installation at https://nodejs.org/en/.
Then open your terminal. Select a path where you want to set up the app and run the command.
npx create-react-app my-react-app
(npx comes with npm 5.2+ and higher, if you are using the old version, see instructions for older npm versions(https://gist.github.com/gaearon/4064d3c23a77c74a3614c498a8bb1c5f))
It will create a folder structure as below;
my-react-app
├── README.md
├── node_modules
├── package.json
├── .gitignore
├── public
│ ├── favicon.ico
│ ├── index.html
│ └── manifest.json
└── src
├── App.css
├── App.js
├── App.test.js
├── index.css
├── index.js
├── logo.svg
└── registerServiceWorker.js
Select your application path by the command;
cd my-react-app
Then run the application using;
npm start or yarn start.
The above process is now completed; you can see the application running in your browser at http://localhost:3000 (default port). Now, we can move to the latest feature introduced by JavaScript called ES6(ECMAScript 2015). Here, are some features that are mostly used in all type of applications.
- let and const
let and const are introduced for declaration in JavaScript.
For Example;
let a = 10;
const a = 10;
In our previous JavaScript, we used var for variable declaration and we do not have a specific structure for our declarations. Some variables are re-assigned and some are not, likewise all the variables are together in a complex manner. But while the introduction of ES6, let and const make them well structured. The variables that need to be changed are declared as let variables, and that are not re-assigned are declared as const. This gives us a perfect structure to differentiate the variables.
- Template literals
This is the simplest feature that can be used to manage the strings along with the expressions.
The syntax is;
`string text`
`string text line 1
string text line 2`
`string text ${expression} string text`
tag `string text ${expression} string text`
Template literals are enclosed by the back-tick (` `). Before introducing ES6, we use ‘+’ operator to concatenate the string and the expressions; also, it can be really complex for the developers if the code is too lengthy.
- Spread and Rest operators
Spread operators are three dots (…) used to manage array literals or object literals.
For example;
function mul(a, b, c) {
return a * b * c;
}
const numbers = [2, 4, 6];
console.log(mul(…numbers));
// output will be 48
————————–
const firstList = [‘pen’, ‘book’, ‘eraser’];
const secondList = [‘bag’, ‘shoes’, ‘pencil’];
console.log([…firstList, …secondList]);
// output will be [“pen”, “book”, “eraser”, “bag”, “shoes”, “pencil”]
Rest operators are also syntactically similar and allow us to represent an indefinite number of arguments as an array.
function school(…args) {
// We can access each node from the args like
console.log(args.name)
console.log(args.address)
}
- Default Parameter Values
ES6 allow function parameters to have default value, if no value or undefined is passed.
For example;
function add(x, y = 10) {
// y is 10 if not passed or undefined
return x + y;
}
console.log(add(5));
// output will be 15
So, there would be no need of matching the parameters. Also, it will be more helpful while working with these kinds of scenarios. These are the majorly used features in ES6 as well as there are lot more features introduced in ES6.