Flutter's Animation Support: How to Add Animations in Flutter?
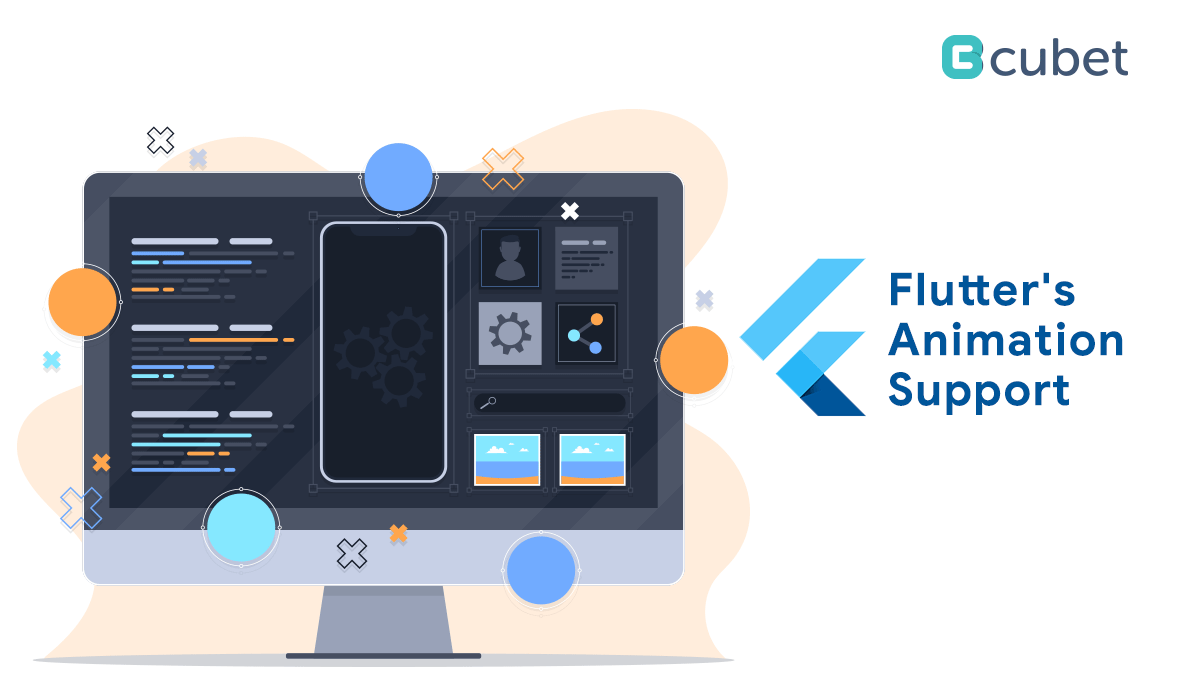
Being a Flutter developer, if you are looking to include some animations in your app and if you feel you are stuck on how to start, this guide will be of great help to you. You will find a variety of animation widgets and various types of animations that are possible in Flutter. With this blog, you will be able to decide which animation will suit the best according to the goals and needs of the project.
Types of Animations in Flutter
- Code-based animations
These animations focus on animating the presently available widgets like row, container, column, stack, etc. You can even change the scale, size, position, etc. of the widget. For example, you can move a product image from the shopping list to the cart icon. With code-based animations in Flutter, you can either use implicit or explicit animations.
- Drawing-based animations
Simple to understand as these animations are used to create animated drawings. Usually, you can do it with a custom painter or any other such animation framework, For eg. Rive.
- Implicit animations with examples
Generally, these types of animations technically fall under code-based animations. They are very simple to implement as compared to other types of animations. What you need to do is create a new value and hit a setState to animate the widget to the new value.
When can you execute the implicit animations in Flutter?
If you want to create a simple animation for any widget, try to find an implicit animated widget on the Flutter website. In case you want to move anything just once, just wrap it inside the AnimatedContainer or AnimatedPositioned.
List of implicit animated widgets for Flutter:
- AnimatedAlign
- AnimatedContainer
- AnimatedOpacity
- AnimatedPadding
- AnimatedPositioning
- AnimatedSize
A small example of Implicit Animation
Suppose you aim to create a rocket that flies when someone clicks the launch button. From a technical aspect, all you need to do is change the position of the rocket the moment the button is clicked.
Wrap the rocket image inside the AnimatedContainer.
duration: Duration(milliseconds: 500),
Stack(
alignment: AlignmentDirectional.bottomCenter,
children: <Widget>[
// Night sky image here
AnimatedContainer(
duration: Duration(milliseconds: 500),
height: 350,
width: 250,
margin: EdgeInsets.only(bottom: bottomMargin),
child: Image.asset(‘assets/images/rocket.png’),
),
// Launch button here
],
)
The duration argument for any implicit widget needs to determine the time to complete the animation. Here in this example, we ask the rocket to reach the top in just a half second and for that, we set the duration parameter to 500 milliseconds.
duration: Duration(milliseconds: 500),
The bottom margin is currently set to the bottomMargin variable, which is 0 when the app starts.
double bottomMargin = 0;
Once the button is clicked, the only thing left is to set the new value. In this case, we’ll increase the bottomMargin to 500:
RaisedButton(
onPressed: () {
_flyRocket();
},
child: Text(
‘Launch’,
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 24),
),
)
void _flyRocket() {
setState(() {
bottomMargin = 500;
});
}
- Explicit animations with examples
They too are a part of code-based animations and are called explicit because you need to start it explicitly. You may find yourself writing code for explicit animations as compared to implicit animations, however, it has its benefits. For example, it can give you more control over the animations that are carried out on a widget.
When can you execute the explicit animations in Flutter?
When you need an animation to run forever or in reverse order, you can use explicit animations. Also, the same can be used when you want to animate multiple widgets similarly. For example, if you want to move something and bring it back to its original position, use SlideTransition to move and AnimationController to get to the starting position.
Here are some examples of explicit animation widgets:
A small example of Explicit animation:
To showcase the concept of explicit animation, we shall use the above example and shall now use a new feature of aborting the flight.
First, add AnimationController and Animation:
Animation<Offset> animation;
AnimationController animationController;
AnimationController is the main actor here; it can control the animation at any given time, such as play, pause, stop, listen to current animation values, etc.
Next, initialize AnimationController and Animation.
Duration is specified while creating the AnimationController.
A tween generates the values between Offset(0, 0) and Offset(0, -1.2). You can create a tween of any object. This gives the higher level flexibility to animate almost any property of the widget.
@override
void initState() {
super.initState();
animationController =
AnimationController(vsync: this, duration: Duration(seconds: 1));
animation = Tween<Offset>(begin: Offset(0, 0), end: Offset(0, -1.2))
.animate(animationController);
}
The next step is to write a widget to animate. SlideTransition is a widget that consumes the animation values.
SlideTransition(
position: animation,
child: Container(
height: 350,
width: 250,
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage(‘assets/images/rocket.png’),
)),
))
Now let’s launch our rocket. The rocket is moved using
animationController.forward();:
RaisedButton(
onPressed: () {
animationController.forward();
},
child: Text(
‘Launch’,
style:
TextStyle(fontWeight: FontWeight.bold, fontSize: 24),
),
color: Colors.red,
textColor: Colors.white,
shape: BeveledRectangleBorder(
borderRadius: BorderRadius.only(
topLeft: Radius.circular(15),
bottomRight: Radius.circular(15))),
)
To make the rocket abort, we’ll bring it back to its starting point using
animationController.reverse();:
RaisedButton(
onPressed: () {
if (animationController.isCompleted) {
animationController.reverse();
}
},
child: Text(
‘Abort’,
style:
TextStyle(fontWeight: FontWeight.bold, fontSize: 24),
),
color: Colors.red,
textColor: Colors.white,
shape: BeveledRectangleBorder(
borderRadius: BorderRadius.only(
topLeft: Radius.circular(15),
bottomRight: Radius.circular(15))),
)
Conclusion
It is important to build apps with thoughtful and well-choreographed animations. The effort in this blog was to explain the differences between code and drawing-based animation. Also, we walked you through the ways of using implicit and explicit animations in your Flutter app. The widgets listed above for each category of animation can also do wonders. Hope this adds great value to your knowledge and based on that we hope you can create compelling animations in your next Flutter project.