Building AI-Powered Web Applications with Laravel
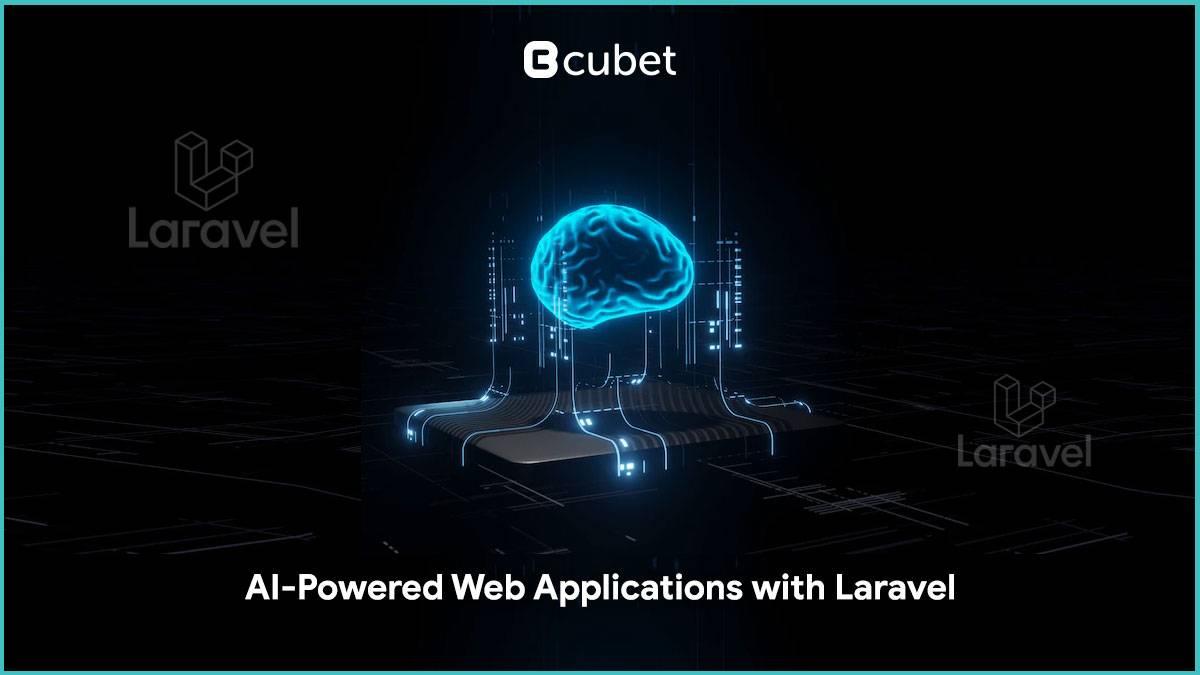
The article highlights the benefits of combining Laravel and AI for web development and the potential of AI in web development. It provides use cases of AI in web development, including natural language processing, image recognition, and predictive analytics, and discusses how they can be integrated into Laravel. The article also provides an overview of best practices for building AI-powered web applications with Laravel and highlights recent AI and machine learning advancements that can be leveraged with Laravel. The conclusion summarizes the key takeaways and the potential benefits of using Laravel and AI together for building web applications.
Introduction
Laravel is a robust open-source web application framework that revolutionizes the development of web applications. There are over 744,615 live websites using Laravel. It was also termed the most desirable framework of 2022.
And why not? Laravel development makes it more accessible, faster, and more efficient. With the recent advancements in Artificial Intelligence (AI), developers can now use Laravel to create robust, complex, intelligent, and efficient web applications.
Use Cases
Leveraging the power of AI, developers can further enhance the capabilities of their applications with intelligent features such as predictive analytics, natural language processing, and machine learning. As the demand for sophisticated user experiences is rising, AI and its range of applications are proving to be a boon for multiple industries.
Here are some common use cases and applications of AI in web development.
1. Natural Language Processing
Natural Language Processing (NLP) is a popular application of AI in web development that can enable users to interact with a web app using natural language instead of pre-defined commands.
This could be done by adding a chatbot to a website, for example, to help guide users through the website or answer questions about the product. Laravel offers various packages and components to implement NLP technologies, such as BotMan and BotMan Studio, making it easy for developers to get started.
2. Image Recognition
This is another widespread use case of AI in web development. This technology can allow a web application to recognize objects in images, such as faces or products. AI-powered applications can improve the user experience by enabling users to search for items based on an image they have taken or to provide automated tagging of pictures uploaded by users. Laravel provides packages and components such as Image Intervention and Image Recognition that make it easy to integrate this technology into a web application.
3. Predictive Analytics
Predictive analysis is used in web applications to identify patterns in user behavior, allowing the app to anticipate user needs better and offer improved user experiences. Laravel provides various packages and components, such as prediction IO and predictive analytics toolkit, that make it easy to integrate predictive analytics into a web app.
In addition, Laravel provides an array of features and services that make it easy for developers to build high-quality backend web development. From authentication and authorization to caching and queuing—Laravel provides everything needed to create robust and powerful web applications with AI technologies.
Laravel Integration with AI
While Laravel is commonly used for web development, it has recently tapped into AI. The framework offers multiple avenues for integrating artificial intelligence (AI) into your applications.
Firstly, Laravel has a comprehensive set of in-built libraries and tools, such as the Eloquent ORM, used to construct AI-driven applications.
Secondly, Laravel can be integrated with cutting-edge AI-based services like TensorFlow and Keras to tap into their advanced capabilities.
The integration process is straightforward and allows developers to use pre-trained models, such as Convolutional Neural Networks (CNNs) and Recurrent Neural Networks (RNNs), or build new models within the TensorFlow and Keras ecosystem. With the integration, developers can utilize TensorFlow’s computational graph and Keras’ high-level API to simplify the process of building and training machine learning models.
In summary, Laravel provides numerous options for integrating AI, both through its in-built libraries and tools and through integration with AI-based services such as TensorFlow and Keras.
Integrating Laravel with Tensorflow
There are a few different ways to do this, but one common approach is to use TensorFlow’s Python library in conjunction with a package like TensorFlow PHP.
Here’s an example of how you might use TensorFlow with Laravel to build an image recognition application:
1. Install TensorFlow PHP: To use TensorFlow in Laravel, you’ll need to install the TensorFlow PHP library. You can install it using Composer:
composer require tensorflow/tensorflow
2. Create a new model: In your Laravel application, create a model responsible for handling the image recognition logic. This model will use TensorFlow’s Python library to perform image recognition.
3. Use the TensorFlow PHP package to interact with the model: You can use the TensorFlow PHP package to interact with the model you created in step 2. For example, the run method can pass an image to the model and get the prediction result.
use TensorFlow\Tensor;
use TensorFlow\Lite\Interpreter;
class ImageRecognitionModel
{
protected $interpreter;
public function __construct()
{
$this->interpreter = new Interpreter(__DIR__ . ‘/path/to/model.tflite’);
}
public function recognize(string $imagePath)
{
$inputTensor = $this->interpreter->getInputTensor(0);
$inputTensor->resize([1, $inputTensor->shape()[1], $inputTensor->shape()[2], $inputTensor->shape()[3]]);
$inputTensor->setFromFile($imagePath);
$this->interpreter->invoke();
$outputTensor = $this->interpreter->getOutputTensor(0);
return $outputTensor->flat();
}
}
- Use the model in your controller: You can use the model in your controller to handle the image recognition logic.
class ImageRecognitionController extends Controller
{
public function recognize(Request $request)
{
$model = new ImageRecognitionModel();
$result = $model->recognize($request->image);
// Do something with the result
}
}
It’s worth noting that TensorFlow PHP is a lightweight library and cannot run heavy computations; it’s meant to be used as a bridge between PHP and Python, and it’s useful for loading models, making predictions, and running inferences.
How to implement NLP using Laravel?
Natural Language Processing (NLP) is a subfield of AI that deals with the interaction between computers and human languages. Here’s an example of how you might use Laravel to implement NLP:
1. Install NLP libraries: First, you’ll need to install an NLP library to use in your Laravel application. There are several popular libraries available, such as NLTK and spaCy. You can install them using Composer:
composer require nltk
composer require spacy
2. Create a new service: In your Laravel application, create a new service that will handle the NLP logic. This service will use the NLP library to perform the NLP tasks.
class NlpService
{
protected $nlp;
public function __construct()
{
$this->nlp = new NLTK();
}
public function processText(string $text)
{
// Perform NLP tasks using the NLTK library
$tokenizedText = $this->nlp->tokenize($text);
$taggedText = $this->nlp->posTag($tokenizedText);
return $taggedText;
}
}
3. Use the service in your controller: You can use the service in your controller to handle the NLP logic.
class NlpController extends Controller
{
public function process(Request $request)
{
$nlpService = new NlpService();
$result = $nlpService->processText($request->text);
// Do something with the result
}
}
How to Implement Image processing using Laravel?
Image Processing is a field of computer science that deals with the manipulation of images. Here’s an example of how you might use Laravel to implement image processing:
1. Install an Image Processing library: First, you’ll need to install an image processing library to use in your Laravel application. There are several popular libraries available, such as Imagine and Intervention Image. You can install them using Composer:
composer require intervention/image
2. Create a new service: In your Laravel application, create a new service that will handle the image processing logic. This service will use the image processing library to perform the image processing tasks.
class ImageProcessingService
{
protected $image;
public function __construct()
{
$this->image = new Image();
}
public function resize(string $imagePath, int $width, int $height)
{
$this->image->make($imagePath)->resize($width, $height)->save();
}
}
3. Use the service in your controller: You can use the service in your controller to handle the image processing logic.
class ImageProcessingController extends Controller
{
public function resize(Request $request)
{
$imageProcessingService = new ImageProcessingService();
$imageProcessingService->resize($request->image, $request->width, $request->height);
// Do something with the result
}
}
Here are a Few Best Practices for Building AI-powered Web Applications with Laravel:
1. Data Preparation: It’s important to prepare your data properly before building your AI model. This includes tasks such as cleaning the data, normalizing the data, and splitting the data into training, validation, and test sets.
2. Model Training: When training your AI model, it’s essential to use a large enough dataset and experiment with different algorithms and configurations to find the best-performing model.
3. Deployment: Consider using cloud-based platforms such as AWS, Azure, or Google Cloud for deploying your AI model. These platforms provide potent tools for deploying, scaling and monitoring your AI models.
4. Model Versioning: It’s essential to version your models keep track of the different versions of your model. This will help you roll back to a previous version of your model if needed.
5. Monitoring: Monitor your AI model’s performance in production by collecting metrics, analyzing logs, and monitoring for errors. This will help you detect any issues early on and make adjustments to improve the model’s performance.
6. Security: Consider security when building AI-powered web applications. This includes tasks such as encrypting sensitive data, securing the communication between the client and the server, and preventing unauthorized access to the model.
7. Ethics: AI models can be used to make predictions, but it’s important to be aware of the ethical considerations and potential biases in the data and to be transparent about the limitations of the model.
By following these best practices, you can ensure that your AI-powered web applications built with Laravel are performant, scalable, and secure.
Advancements
In the past few years, rapid advancements in artificial intelligence and machine learning have revolutionized web apps and backend web development. These advances can be easily leveraged with Laravel, designed to make backend web development a breeze.
From helping developers craft powerful algorithms to developing smart search engines, Laravel provides developers with the tools to create sophisticated, AI-powered web applications.
The new capabilities, developers can use Laravel to build more advanced web applications to anticipate user needs, analyze data better, and provide personalized experiences. For example, a music streaming service could leverage machine learning to deliver personalized recommendations based on user listening habits. AI-powered applications built with Laravel can also provide enhanced security features such as facial recognition and automated detection of malicious activities.
In addition, Laravel can help developers quickly create intelligent chatbots and voice assistants. These AI-driven tools can provide customer support, generate insights from large datasets, and even automate specific processes.
Conclusion
Laravel is a robust and reliable framework for building web applications, and it has become even more powerful with the integration of AI. With its simple syntax, robust tools, and dedicated support, Laravel is an ideal platform for creating AI-powered web applications.
At Cubet, we understand the importance of staying at the forefront of technology and its applications in web development. As such, we are proud to present the benefits of utilizing Laravel and Artificial Intelligence (AI) in tandem for building cutting-edge web applications.
Leveraging AI in web development opens up a multitude of possibilities and use cases.
We believe in following best practices to ensure successful AI-powered web applications. These best practices include data preparation, model training, and deployment. Our team of experts will work with you to ensure that your project adheres to these guidelines and yields optimal results.
Furthermore, Cubet is excited to highlight the recent advancements in AI and machine learning that are applied to web development. These advancements can be leveraged with Laravel to bring your web application to new heights.
In conclusion, Cubet is confident in the potential benefits of utilizing Laravel and AI together in web development. Our team of experts will work with you to bring your vision to life, leveraging the latest technology and best practices to achieve exceptional results.