Let's Discuss Laravel's Invokable Custom Validation Rules
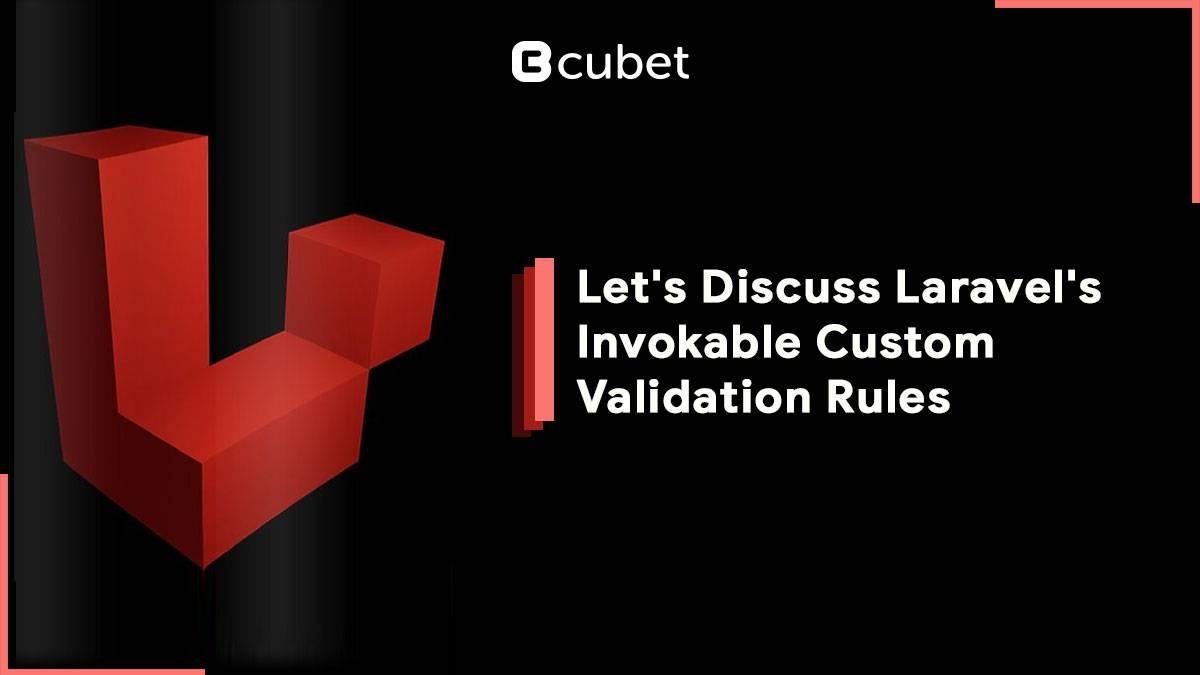
So you’ve used custom validation rules in Laravel before. They’re an excellent way to add additional validation checks to your user input that don’t fit under any existing rule. But what if you want to take things one step further? Let’s say, for example, that you have an Invokable class and want to check it when validating input parameters. You could write a condition like this: pre>
But is this the optimal solution? Probably not. So let’s discuss how we can improve our implementation of custom validation rules in Laravel by using the Invokable trait and its all() method.
What is custom validation?
Custom validation is a feature that allows you to define a set of rules for a custom field. The rules can be based on various factors, including the field’s type, the field’s data type, and the field’s context.
Custom validation can also prevent specific errors when submitting a custom field. For example, you might want to avoid an error if the custom field’s value is empty or if the custom field’s value is too long.
Custom validation can also provide additional security when using a custom field. For example, you might want to require that a custom field’s value be provided in an encrypted form or that it be provided only by a specific user.
Custom Validation in Laravel
Validation in Laravel is a powerful tool that can be used to ensure that your application’s inputs are valid before they are processed. Validation is essential regarding user-entered data, but it can also be useful for fields that require special attention, such as passwords and credit card numbers.
When using validation, you should always start by defining what kind of validation you want to perform. This will help you decide which fields should be checked and how you should do it. You can define validation rules with simple expressions or use custom validation rules with the validation methods provided by Laravel.
When defining your rules, you should consider the following:
- What kind of validation is being applied? For example, is it required or optional? Is it case-sensitive?
- How should the value of the field be validated? Should it be compared to another value (such as a minimum or maximum value) or just tested against a given set of conditions?
- What other fields should be included in the scope of the rule? For example, if you are checking a date field and your date must be between two dates, you must ensure that all other fields on the form are also within those dates.
Once you have defined your rules, you can use them to validate user input. Validation errors will appear at the top of the
Invokable Validation Rules
Validation in Laravel is a powerful way for your application to verify data before it’s stored. It can be used for any number of purposes, from restricting access to sensitive fields to ensuring that data conforms to a particular schema.
When using validation, it’s essential to understand the different types available in Laravel:
- Element-level validation:
Elements can be validated by setting custom rules for them. For example, if you wanted to ensure that all user names were unique within the system, you could use the laravel:unique@id> rule.
- Model-level validation:
This type of validation applies to the entire model instead of individual fields. This allows you to validate that a whole object meets your requirements (e.g., all lots are valid).
- Global validation rules:
These rules apply across the entire application and can be set per route or controller. They are run at the time of request validation, so you should define them in your routes or controllers where they will be most helpful.
Strong Validation Rules
Validation rules are strict conditional statements that tell the application what data is valid to enter and what is not. Validation rules ensure that data entered belongs to the table or belongs to a specific field (or combination of fields). It can validate data type, range, length, email address, and other properties.
Like validation rules in other programming languages, laravel validation rules can be defined in model files. These rules may be tested against the model at runtime for validation errors.
The validation rules are defined in an array inside your app/models/ rule array. This array contains all the constraints used when you try to populate your model with data (including validations).
When invalid data enter your model, the actions that can be taken are specified as methods of the Rule class. The available actions include:
Reject: Return false if any of the specified conditions are not met. A custom HTTP Server middleware usually does this.
Return false if any of the specified conditions are not met. A custom HTTP Server middleware Skip usually does this: Skip over this rule entirely and continue parsing the model. This allows you to override a rule with no side effects when the data meets specific criteria (for example, when you only want certain fields populated)
Skip over this rule entirely and continue parsing the model.
Conclusion
You can use custom validation rules in your Invokable classes to validate data before storing it. This article will validate data using the Invokable trait and its all() method.
To use custom validation rules in your Invokable class, you will need to define your rules in a file in your app/models directory and implement the Validation trait.
Once you have defined your rules and implemented the Validation trait in your class, you can use them to validate user input. Validation errors will appear at the top of the page when a validation error occurs.
The validation rules that you defined in your model file (which is, by default, app/models/Rule.php) will be applied to the validated input. You can also use the all() method to test input against the rules defined in your model.
Note that you can also use the InputValidatorService provided by Laravel to validate user input.